Introduction
Grocery store data provides invaluable insights into market trends, product availability, pricing strategies, and customer preferences. Businesses increasingly rely on Grocery Store Data Scraping Services to stay competitive in the food retail industry. In this detailed guide, we’ll explore How to Scrape Grocery Store Data, provide step-by-step instructions, share practical examples, and include the necessary coding snippets for implementation. Additionally, we’ll cover how to Extract Grocery Store Product Data and Extract Grocery Store Location Data, empowering businesses, data analysts, and developers to gain deeper insights into grocery stores' product offerings, pricing, and geographical reach.
Importance of Grocery Store Data Scraping
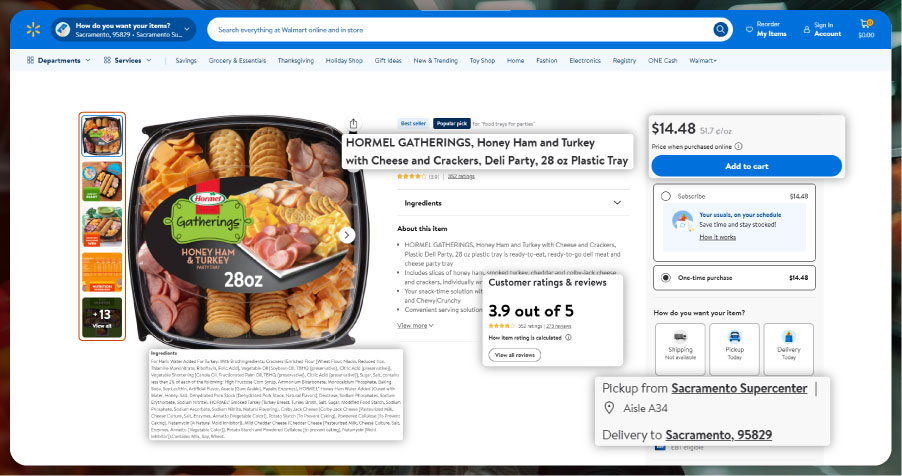
Grocery store data scraping plays a crucial role in the modern retail and e-commerce landscape. By extracting valuable information from grocery store websites, businesses gain insights into product availability, pricing strategies, customer preferences, and regional market trends. This data is essential for staying competitive and enhancing operational efficiency in an increasingly data-driven world.
One of the most significant advantages of grocery store data scraping is Web Scraping Grocery Store Product Prices Data, which enables real- time price tracking. Retailers can monitor competitors' prices and adjust their own pricing strategies accordingly, ensuring they remain competitive while maximizing profit margins. Additionally, scraping data helps businesses track product availability, preventing stockouts and ensuring they meet customer demand.
Another important aspect is customer behavior analysis. Using tools like Grocery Store Product Data Scraping API, businesses can scrape product reviews, ratings, and feedback to gain a deeper understanding of what customers value in terms of quality, pricing, and product features. By analyzing these patterns, businesses can Extract Supermarket Product Information, optimize their product offerings, and improve their overall customer experience.
Grocery store data scraping also aids in market expansion by extracting Grocery Store Product Prices Data and location-specific information, helping companies identify underserved regions or trends. Furthermore, businesses can Scrape Grocery Pricing and Product Data or Scrape Supermarket Product Data to leverage data intelligence tools for personalized marketing strategies and pricing optimization, ultimately boosting sales and customer retention.
Through comprehensive Grocery Store Product Prices Data Extraction, businesses can stay ahead in the competitive retail and e-commerce market by harnessing actionable insights.
Applications of Grocery Store Data Scraping
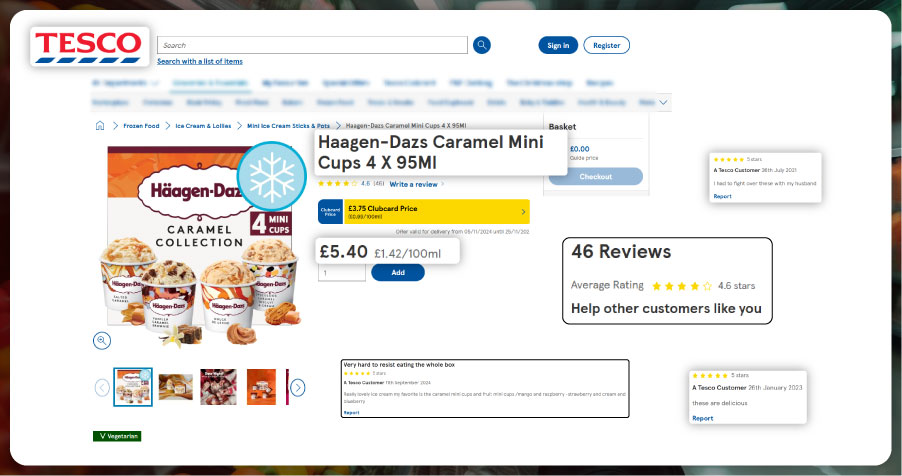
Grocery store data scraping offers a wide range of applications that can significantly enhance business strategies across various sectors. One of the key applications is competitive pricing analysis. By scraping real- time price data from competitors’ grocery websites using tools like a Grocery Delivery Data Scraping API, businesses can monitor pricing trends, identify underpriced or overpriced items, and adjust their own pricing strategies to stay competitive. This enables better price optimization and maximizes profit margins.
Another important application is inventory and stock management. By tracking product availability and stock levels across multiple grocery stores, businesses can gain insights into which items are in demand and ensure that their own inventory is aligned with market trends. This helps avoid stockouts and ensures that customers can always find what they need. Leveraging Grocery Delivery Datasets for inventory insights enhances supply chain efficiency and customer satisfaction.
Customer behavior analysis is also a major application of grocery data scraping. By extracting product reviews and ratings, businesses can gain insights into consumer preferences, helping them identify which products are most popular and why. This information can be used to fine-tune product offerings, marketing strategies, and promotional efforts.
Additionally, regional market insights can be gained by scraping location-specific data. Businesses can Extract Grocery Store Location Data to identify regional pricing differences, customer preferences, and trends. This application is particularly useful for businesses looking to expand into new geographical areas and optimize their operations.
Furthermore, Grocery Pricing Data Intelligence allows businesses to harness the power of data-driven decision-making, enabling dynamic pricing strategies, identifying emerging market trends, and improving overall competitiveness in the grocery retail industry.
Tools for Grocery Store Data Scraping
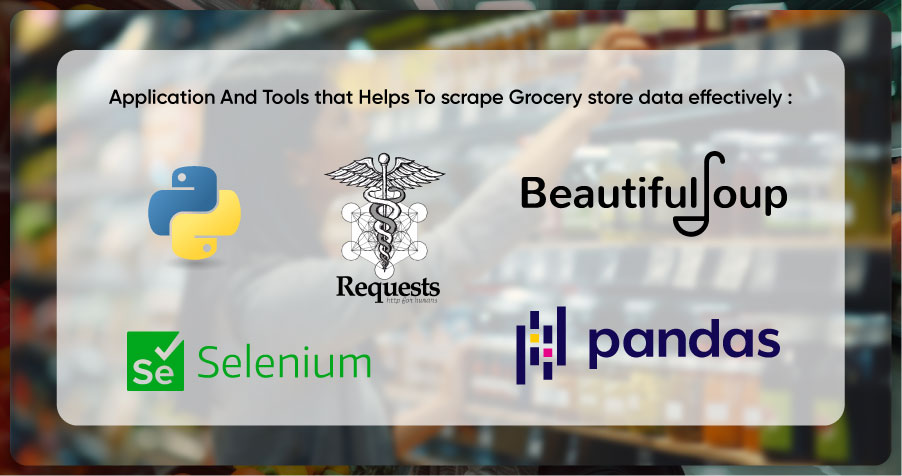
To scrape grocery store data effectively, you’ll need:
Python: The primary programming language for web scraping.
BeautifulSoup: For HTML parsing and navigation.
Selenium: To handle dynamically loaded content.
Pandas: For data organization and analysis.
Requests: For fetching webpage HTML content.
Install the necessary libraries using pip:
pip install requests beautifulsoup4 selenium pandas
Step-by-Step Guide to Scraping Grocery Store Data
Step 1: Analyze the Target Website
Visit the grocery store website and identify the structure of the pages containing the required information. Key areas to focus on include:
- Product details: name, price, category, and description.
- Pagination or infinite scrolling.
- HTML elements associated with these data points.
Step 2: Set Up Your Environment
Install Python and the necessary libraries. Create a project folder to store your scripts and data outputs.
Step 3: Fetch and Parse HTML Content
import requests
from bs4 import BeautifulSoup
url = "https://www.examplegrocerystore.com/products"
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)
AppleWebKit/537.36 (KHTML, like Gecko) Chrome/117.0.0.1
Safari/537.36"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify()) # View the page structure
Begin by fetching the webpage's content using the Requests library.
Step 4: Extract Product Information
products = []
for item in soup.find_all('div', class_='product-card'):
product_name = item.find('h2', class_='product-title').text.strip()
product_price = item.find('span', class_='price').text.strip()
availability = item.find('div', class_='availability').text.strip()
products.append({
'Product Name': product_name,
'Price': product_price,
'Availability': availability
})
# Preview extracted data
for product in products[:5]:
print(product)
Identify the HTML tags containing the product name, price, and availability.
Step 5: Handle Pagination
base_url = "https://www.examplegrocerystore.com/products?page="
for page in range(1, 11): # Adjust range based on the number of pages
response = requests.get(base_url + str(page), headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
for item in soup.find_all('div', class_='product-card'):
product_name = item.find('h2', class_='product-title').text.strip()
product_price = item.find('span', class_='price').text.strip()
availability = item.find('div', class_='availability').text.strip()
products.append({
'Product Name': product_name,
'Price': product_price,
'Availability': availability
})
If the website uses pagination, modify the script to scrape data from multiple pages.
Step 6: Handle Infinite Scrolling
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
driver.get("https://www.examplegrocerystore.com/products")
while True:
driver.execute_script("window.scrollTo(0,
document.body.scrollHeight);")
time.sleep(2) # Wait for the content to load
try:
load_more_button = driver.find_element(By.CLASS_NAME, "load-
more")
load_more_button.click()
except:
print("No more pages to load.")
break
soup = BeautifulSoup(driver.page_source, 'html.parser')
driver.quit()
For websites with infinite scrolling, use Selenium.
Step 7: Organize and Export Data
import pandas as pd
df = pd.DataFrame(products)
df.to_csv('grocery_data.csv', index=False)
print("Data saved successfully!")
Use Pandas to save the data into a structured format like CSV.
Use Cases
Grocery store data scraping offers businesses the opportunity to leverage market insights, enhance customer experiences, and improve operational efficiency across various use cases. Below are some key use cases of grocery store data scraping:
Competitive Price Monitoring
One of the most valuable use cases for grocery store data scraping is competitive price monitoring. By extracting pricing data from competitor websites, businesses can track fluctuations in product prices and adjust their own pricing strategies accordingly. This allows businesses to remain competitive, optimize their prices for higher sales, and strategically respond to price changes in the market. With Grocery Store Product Price Data Scraping, businesses can automate the tracking of key products, ensuring their prices are always aligned with market trends.
Inventory and Stock Optimization
Scraping data helps businesses track product availability across grocery stores, providing insights into which products are in high demand and which are underperforming. This can be crucial for businesses looking to optimize their inventory management and avoid stockouts or overstocking. With real-time data extraction, businesses can adjust their inventory levels accordingly, ensuring they meet customer demand while maintaining cost efficiency.
Customer Behavior Insights
By scraping product reviews, ratings, and other customer feedback, businesses can gain valuable insights into customer behavior. Analyzing reviews and ratings can help identify customer preferences, satisfaction levels, and pain points, providing businesses with data to optimize product offerings. This helps create personalized marketing strategies, improve product development, and enhance customer experience.
Market Expansion and Regional Analysis
Location-specific scraping allows businesses to analyze regional trends, pricing, and preferences across different markets. For example, if a grocery store is expanding into a new region, scraping Grocery Store Location Data can provide insight into local pricing strategies, popular products, and customer needs, enabling businesses to tailor their approach and enter the market with a more informed strategy.
Price Intelligence and Strategy
Price intelligence is another critical use case of grocery store data scraping. By tracking the pricing strategies of competitors, businesses can implement dynamic pricing models, introduce discounts, or optimize product pricing to increase their market share. This data also helps businesses track seasonal pricing changes, detect promotional trends, and adapt their own pricing strategies.
Grocery Delivery Optimization
Grocery delivery companies can use grocery store data scraping APIs to track product availability and pricing in real-time. By monitoring competitor pricing, delivery fees, and product availability, delivery services can adjust their logistics and pricing strategies to remain competitive while ensuring timely deliveries.
These diverse use cases showcase how grocery store data scraping can be a powerful tool for driving business growth, improving customer satisfaction, and staying ahead in an increasingly competitive market. By leveraging data scraping, businesses can access real-time, actionable insights that help them make better-informed decisions.
Case Studies of Grocery Store Data Scraping
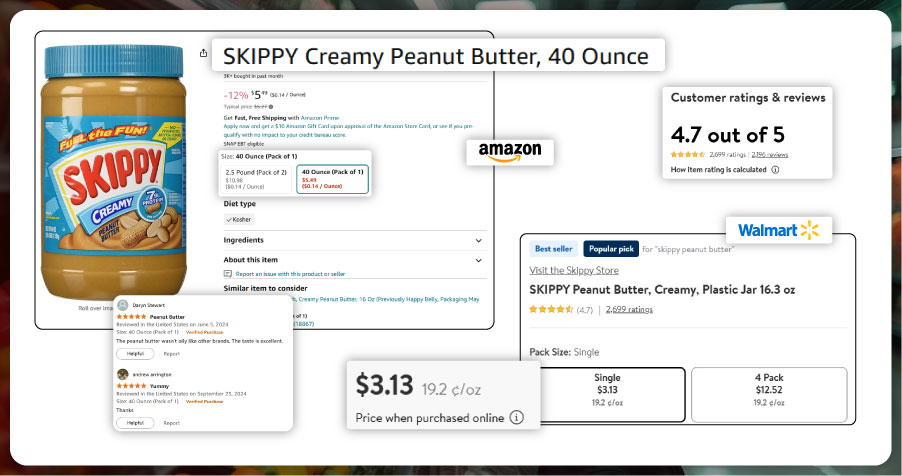
Here are a few real-life case studies that illustrate how grocery store data scraping has been successfully applied to drive business success and growth across various sectors:
1. Competitive Pricing Strategy for an E-commerce Grocery Retailer
A leading online grocery retailer faced challenges with staying competitive against larger market players. They used Grocery Store Data Scraping to monitor pricing data from several competitors, including major supermarket chains and online grocery services. By scraping product prices in real-time, the retailer was able to make rapid adjustments to their pricing strategy, ensuring that they remained competitive while maintaining healthy profit margins. The real-time data enabled them to spot trends and adjust prices for high-demand products such as fresh produce and organic food. This strategy not only boosted their sales but also helped increase customer loyalty, as shoppers recognized the retailer’s consistently competitive prices.
2. Inventory Management for a Local Grocery Store Chain
A regional grocery store chain with multiple locations was struggling to manage stock levels efficiently. By implementing Grocery Store Product Data Scraping, the chain was able to extract real-time information on product availability from competitor websites and larger supermarkets. The data provided insights into which products were frequently out of stock and which were consistently popular. By leveraging this data, the grocery chain could better forecast demand and optimize their inventory, reducing stockouts and overstocking issues. This resulted in improved operational efficiency, reduced waste, and enhanced customer satisfaction due to better product availability.
3. Customer Behavior Analysis for a Grocery Delivery Service
A grocery delivery service used Web Scraping Grocery Store Product Prices Data to track customer reviews, product ratings, and purchase patterns across multiple grocery websites. By analyzing this data, they identified which product categories were most popular among customers and understood the factors influencing purchasing decisions, such as price, quality, and delivery times. With this information, the service was able to tailor their offerings to match customer preferences, optimize their product catalog, and personalize marketing campaigns. This led to a significant increase in sales and customer retention as they were able to meet the growing demand for specific products while maintaining competitive pricing.
4. Regional Market Analysis for a National Supermarket Chain
A national supermarket chain looking to expand its presence into a new geographic region used Grocery Store Location Data Scraping to gather insights into local market dynamics. They scraped data from local competitors to understand regional pricing strategies, popular product categories, and customer preferences in the new location. By analyzing this data, they were able to tailor their product selection and pricing to meet the specific needs of the local population. As a result, the supermarket chain successfully launched its new stores, gaining market share faster than expected due to a more targeted and informed approach.
5. Dynamic Pricing Implementation for an Online Grocery Platform
An online grocery platform used Grocery Price Tracking Dashboard powered by data scraping to implement dynamic pricing. They scraped competitor pricing, promotional offers, and market trends to adjust their prices in real-time. For instance, when a competitor introduced a flash sale on specific products, the platform quickly adjusted its prices to offer competitive discounts without compromising on profit margins. This approach not only improved their sales during peak times but also allowed the platform to maintain its reputation for offering competitive pricing across a wide range of products.
These case studies demonstrate how grocery store data scraping can be a game changer for businesses in the grocery and retail sectors, providing valuable insights into competitive pricing, inventory management, customer behavior, and market expansion. By using scraping technologies, companies can gain a significant competitive edge, enhance operational efficiency, and improve overall customer satisfaction.
Challenges and Solutions in Grocery Store Data Scraping
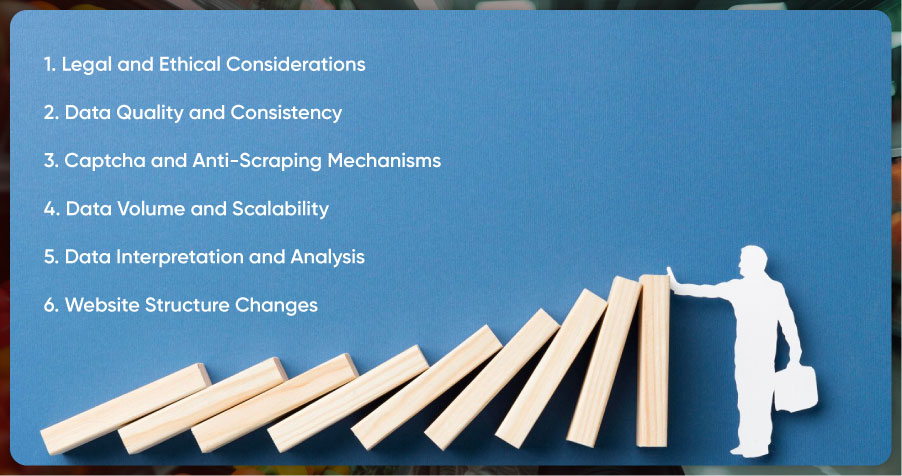
While grocery store data scraping offers valuable insights and opportunities for business growth, there are several challenges that organizations may face when implementing it. Here are some common challenges and potential solutions:
1. Legal and Ethical Considerations
Challenge: One of the biggest challenges with grocery store data scraping is navigating the legal and ethical concerns associated with scraping data from websites. Many websites have terms of service that explicitly prohibit scraping, and businesses need to be cautious about violating these terms, which can lead to legal repercussions or being blocked from accessing the site.
Solution: To mitigate these risks, businesses should ensure they comply with legal requirements and respect robots.txt files, which indicate the rules for web crawlers. It’s important to review the legal framework around data scraping in different regions and secure necessary permissions or agreements with websites. Additionally, adopting ethical scraping practices, such as not overburdening the website’s servers and scraping only publicly available data, can help avoid legal issues. Partnering with professional data scraping service providers can also ensure adherence to compliance regulations.
2. Data Quality and Consistency
Challenge: The quality and consistency of the data extracted from grocery websites can be an issue. For example, product descriptions, pricing, and availability may not be consistently formatted, making it difficult to standardize and analyze the data.
Solution: To overcome this challenge, businesses can use data cleaning techniques to standardize and normalize the data once it's scraped. Advanced scraping tools and machine learning algorithms can be employed to handle inconsistent formats, ensuring that the data is clean, structured, and ready for analysis. Additionally, integrating API data extraction alongside traditional scraping can enhance data accuracy and consistency by pulling structured data directly from sources.
3. Captcha and Anti-Scraping Mechanisms
Challenge: Many websites, including grocery store platforms, employ captcha systems or other anti-scraping mechanisms to prevent automated data extraction. These systems can block scrapers or require human intervention, making large-scale scraping difficult.
Solution: Businesses can use advanced scraping techniques that bypass captchas and anti-scraping barriers, such as using proxy servers, rotating IPs, or incorporating headless browsers. These techniques help mimic human browsing behavior and reduce the chances of being blocked. Furthermore, employing API-based scraping solutions can help avoid these barriers by working within the terms set by the website.
4. Data Volume and Scalability
Challenge: Collecting large volumes of data from multiple grocery websites can be resource-intensive. Ensuring that the data scraping solution can scale efficiently while maintaining speed and accuracy is a common challenge.
Solution: The solution lies in cloud-based scraping platforms and distributed scraping technologies that can handle large-scale operations efficiently. These platforms can handle large amounts of data scraping without compromising performance, and they allow businesses to scale their scraping processes as needed. Automating the entire process with scraping bots and integrating it with cloud-based storage ensures that businesses can collect data from multiple grocery websites concurrently.
5. Data Interpretation and Analysis
Challenge: After collecting data, businesses may struggle with interpreting and analyzing it effectively, especially when dealing with vast amounts of raw, unstructured data.
Solution: To address this, businesses can use data analytics platforms or partner with data science professionals who specialize in interpreting complex datasets. Advanced tools and machine learning algorithms can be implemented to extract actionable insights from the scraped data. For example, data visualization dashboards and predictive analytics can help businesses understand patterns in customer behavior, pricing trends, and inventory levels.
6. Website Structure Changes
Challenge: Grocery websites may frequently update or change their structure, which can break scraping scripts and lead to errors or incomplete data extraction.
Solution: Businesses can mitigate this issue by implementing scraping solutions with built-in adaptability. These solutions can quickly adjust to changes in website structure, ensuring continuous and error-free data extraction. Regular scraping script updates and monitoring of website changes will also help businesses stay ahead of potential disruptions.
In conclusion, while grocery store data scraping offers immense benefits, it is not without its challenges. By implementing best practices and leveraging advanced scraping techniques, businesses can effectively overcome these obstacles and gain valuable insights from grocery store data, ultimately enhancing their market strategies and operational efficiency.
Conclusion
Scraping grocery store data is essential for businesses aiming to optimize pricing, inventory, and customer satisfaction. From competitive analysis to delivery optimization, the applications are endless.
At Food Data Scrape, we offer professional solutions for Grocery Store Data Scraping Services, including real-time APIs and customizable dashboards. Whether you need to build a Grocery Dashboard or extract pricing data, we’re here to help.
Contact Food Data Scrape today and unlock the potential of grocery data for your business success!